The following implementation is a simple example code to integrate an Xbox360 controller into a C-based program. It reads the values of the controller axis and the buttons and displays them on the screen. The following picture shows the output of the code:
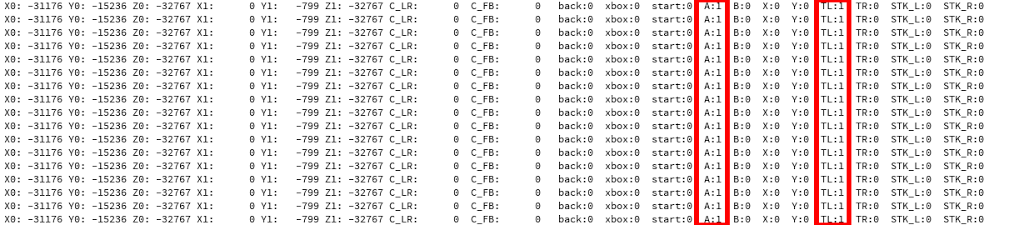
In this case, the buttons „A“ and „TL“, which is the top-left button, were pressed. The project is called xboxControllerClient can be downloaded from my repository at https://github.com/msch26/scholtyssek-blogspot.
The whole configuration is in the header file xboxController.h. There the mapping of the buttons and the axis is implemented. The data will be stored in a struct called xboxCtrl. It is also is in the header file. The following example shows how to use the code:
#include
#include "xboxController.h"
int main(int argc, char **argv) {
if (initXboxContoller(XBOX_DEVICE) >= 0) {
xboxCtrl* xbox = getXboxDataStruct();
readXboxControllerInformation(xbox);
printf("xbox controller detected axis:\t\t%d\nbuttons:\t%d\nidentifier:\t%s\n",
xbox->numOfAxis, xbox->numOfButtons, xbox->identifier);
while (1) {
readXboxData(xbox);
printXboxCtrlValues(xbox);
}
deinitXboxController(xbox);
}
return 0;
}
The method initXboxContoller(XBOX_DEVICE) opens a connection to the device /dev/input/js0. This device is set as the default device. To change this, change the value of XBOX_DEVICE. Next, the method getXboxDataStruct() returns a pointer to the data stuct xboxCtrl. In this struct, all information is stored. You can update the information with a periodic call of readXboxData(xbox). To print the state of the Xbox controller, you should call printXboxCtrlValues(xbox).